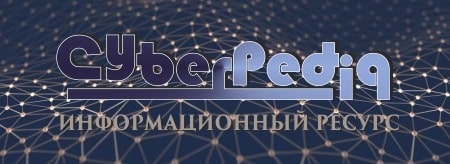
Своеобразие русской архитектуры: Основной материал – дерево – быстрота постройки, но недолговечность и необходимость деления...
Состав сооружений: решетки и песколовки: Решетки – это первое устройство в схеме очистных сооружений. Они представляют...
Топ:
Оценка эффективности инструментов коммуникационной политики: Внешние коммуникации - обмен информацией между организацией и её внешней средой...
Процедура выполнения команд. Рабочий цикл процессора: Функционирование процессора в основном состоит из повторяющихся рабочих циклов, каждый из которых соответствует...
Эволюция кровеносной системы позвоночных животных: Биологическая эволюция – необратимый процесс исторического развития живой природы...
Интересное:
Как мы говорим и как мы слушаем: общение можно сравнить с огромным зонтиком, под которым скрыто все...
Берегоукрепление оползневых склонов: На прибрежных склонах основной причиной развития оползневых процессов является подмыв водами рек естественных склонов...
Что нужно делать при лейкемии: Прежде всего, необходимо выяснить, не страдаете ли вы каким-либо душевным недугом...
Дисциплины:
![]() |
![]() |
5.00
из
|
Заказать работу |
|
|
using System;
namespace Матрица_1_0
{
class AT
{
public void ATR(int ind, Cache ch)
{
int a=0, b=0;
double[,] matr=new double[a,b];
if (ind == 1)
{
a = ch.c;
b = ch.d;
matr = ch.matr1;
}
if (ind==2)
{
a = ch.e;
b = ch.f;
matr = ch.matr2;
}
if (ind == 3)
{
a = ch.g;
b = ch.h;
matr = ch.res;
}
double[,] res = new double[b, a];
for (int i = 0; i < a; i++)
{
for (int j = 0; j < b; j++)
{
res[j,i] = matr[i, j];
}
}
ch.g = b;
ch.h = a;
ch.res = res;
}
}
}
Класс умножения матрицы на число k (Axk.cs)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Матрица_1_0
{
class Axk
{
public void Axk2(int ind, double k, Cache ch)
{
int a = 0, b = 0;
double[,] matr = new double[a, b];
if (ind == 1)
{
a = ch.c;
b = ch.d;
matr=ch.matr1;
}
if (ind == 2)
{
a = ch.e;
b = ch.f;
matr = ch.matr2;
}
if (ind == 3)
{
a = ch.g;
b = ch.h;
matr = ch.res;
ch.res = null;
}
double[,] res = new double[a, b];
for (int i = 0; i < a; i++)
{
for (int j = 0; j < b; j++)
{
res[i, j] = Math.Round(matr[i, j]*k, 3);
}
}
ch.g = a;
ch.h = b;
ch.res = res;
}
}
}
Класс получения канонического вида и ранга матрицы (Kan.cs)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Матрица_1_0
{
class Kan
{
public void KV(int ind, Cache ch)
{
int a=0,b=0,n=0;
int uchet = 0;
double[,] matr=new double[a,b];
if (ind==1)
{
a=ch.c;
b=ch.d;
matr=ch.matr1;
}
if (ind == 2)
|
{
a=ch.e;
b=ch.f;
matr=ch.matr2;
}
q:
if (n < a && n < b)
{
double del = matr[n, n];
if (del!= 0)
{
double[] str = new double[b - n];
double umn = 0;
for (int i = n; i < b; i++)
{
matr[n, i] = matr[n, i] / del;
str[i - n] = matr[n, i];
}
for (int i = n + 1; i < a; i++)
{
umn = matr[i, n];
for (int j = n; j < b; j++)
{
matr[i, j] = matr[i, j] - str[j - n] * umn;
}
}
for (int i = n + 1; i < b; i++)
{
matr[n, i] = 0;
}
for (int i = n; i < a; i++)
{
for (int j = n; j < b; j++)
{
if (i == n || j == n)
{
if (matr[i, j] == 0)
{
uchet++;
}
}
}
}
}
}
if (uchet == ((a + b) - 2)-2*n && uchet!=0)
{
uchet=0;
n++;
goto q;
}
else
{
ch.g = a;
ch.h = b;
ch.res = matr;
}
}
public int Rang(int ind, Cache ch)
{
KV(ind, ch);
int rang = 0;
int n = ch.g;
int m = ch.h;
q:
int vrem = 0;
for (int i = 0; i < n; i++)
{
for (int j = 0; j < m; j++)
{
if (i == n - 1 && ch.res[i,j]==0)
{
vrem++;
}
}
}
if (vrem == m)
{
n--;
goto q;
}
else
{
rang = n;
}
return rang;
}
}
}
Класс чтения матриц из файла (Re.cs)
using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
using System.Windows;
using Microsoft.Win32;
namespace Матрица_1_0
|
{
class RE
{
public int a = 0, b;
public void Read(int ind, Cache ch)
{
Encoding en = Encoding.GetEncoding(1251);
char[] ca = { ' ', ','};
string[] strarray;
string strline;
List<string> mas = new List<string>();
Stream myStream = null;
OpenFileDialog openFileDialog1 = new OpenFileDialog();
openFileDialog1.InitialDirectory = "c:\\";
openFileDialog1.Filter = "matr files (*.matr)|*.matr|All files (*.*)|*.*";
openFileDialog1.FilterIndex = 1;
openFileDialog1.RestoreDirectory = true;
int vrem = 0;
if (openFileDialog1.ShowDialog()!= null)
{
try
{
if ((myStream = openFileDialog1.OpenFile())!= null)
{
using (myStream)
{
StreamReader sr = new StreamReader(myStream, en);
strline = sr.ReadLine();
int k = 0;
while (strline!= null)
{
strarray = strline.Split(ca);
b = strarray.Length;
mas.Add(strline);
strline = sr.ReadLine();
if (k == 0)
{
vrem = b;
if (vrem < 3)
{
string[] strarray2 = mas[0].Split(ca);
ch.name = strarray2[0];
ch.fam = strarray2[1];
a--;
k++;
}
else
{
ch.name = "Автор";
ch.fam = "отсутствует";
k++;
}
}
a++;
}
}
myStream.Close();
}
}
catch
{
MessageBox.Show("Ошибка! Вы не выбрали файл.");
}
}
int l = 0;
double[,] matr = new double[a, b];
for (int i = 0; i < a+l; i++)
{
if (vrem < 3&&l==0)
{
i = 1;
l++;
}
strarray = (mas[i].Split(ca));
for (int j = 0; j < b; j++)
{
if (vrem < 3)
|
{
matr[i - 1, j] = Convert.ToDouble(strarray[j]);
}
else
{
matr[i, j] = Convert.ToDouble(strarray[j]);
}
}
}
if (ind == 1)
{
ch.c = a;
ch.d = b;
ch.matr1 = matr;
}
if (ind == 2)
{
ch.e = a;
ch.f = b;
ch.matr2 = matr;
}
}
}
}
Класс записи результатов вычислений в файл (WR.cs)
using System;
using System.Text;
using System.IO;
using System.Windows;
using Microsoft.Win32;
namespace Матрица_1_0
{
class WR
{
public void Save(Cache ch)
{
SaveFileDialog save = new SaveFileDialog();
save.InitialDirectory = "c:\\";
save.Filter = "matr files (*.matr)|*.matr|All files (*.*)|*.*";
save.FilterIndex = 1;
save.RestoreDirectory = true;
if (save.ShowDialog() == true)
{
string[] zap = new string[ch.g+1];
for (int i = 0; i < ch.g+1; i++)
{
string add=null;
for (int j = 0; j < ch.h; j++)
{
if (i == 0)
{
add = ch.name2 + " " + ch.fam2;
}
else
{
if (j == ch.h - 1)
{
add += ch.res[i - 1, j].ToString();
}
else
{
add += (ch.res[i - 1, j].ToString() + " ");
}
}
}
zap[i] = add;
}
Encoding en=Encoding.GetEncoding(1251);
File.WriteAllLines(save.FileName, zap, en);
}
}
}
}
Класс произведения матриц (Umn.cs)
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
namespace Матрица_1_0
{
class Umn
{
public void Umn2(Cache ch)
{
if (ch.d == ch.e)
{
double[,] matr = new double[ch.c, ch.f];
ch.g = ch.c;
ch.h = ch.f;
for (int i = 0; i < ch.c; i++)
{
for (int j = 0; j < ch.f; j++)
{
for (int k = 0; k < ch.d; k++)
{
matr[i, j] += ch.matr1[i, k] * ch.matr2[k, j];
}
}
}
ch.res = matr;
|
}
else
{
MessageBox.Show("Ошибка! Количество столбцов и строк в матрицах не совпадает.");
}
for (int i = 0; i < ch.g; i++)
{
for (int j = 0; j < ch.h; j++)
{
ch.res[i, j] = Math.Round(ch.res[i, j]);
}
}
}
}
}
|
|
Двойное оплодотворение у цветковых растений: Оплодотворение - это процесс слияния мужской и женской половых клеток с образованием зиготы...
Поперечные профили набережных и береговой полосы: На городских территориях берегоукрепление проектируют с учетом технических и экономических требований, но особое значение придают эстетическим...
Историки об Елизавете Петровне: Елизавета попала между двумя встречными культурными течениями, воспитывалась среди новых европейских веяний и преданий...
Индивидуальные очистные сооружения: К классу индивидуальных очистных сооружений относят сооружения, пропускная способность которых...
© cyberpedia.su 2017-2024 - Не является автором материалов. Исключительное право сохранено за автором текста.
Если вы не хотите, чтобы данный материал был у нас на сайте, перейдите по ссылке: Нарушение авторских прав. Мы поможем в написании вашей работы!