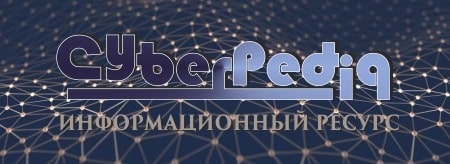
Организация стока поверхностных вод: Наибольшее количество влаги на земном шаре испаряется с поверхности морей и океанов (88‰)...
История развития хранилищ для нефти: Первые склады нефти появились в XVII веке. Они представляли собой землянные ямы-амбара глубиной 4…5 м...
Топ:
Отражение на счетах бухгалтерского учета процесса приобретения: Процесс заготовления представляет систему экономических событий, включающих приобретение организацией у поставщиков сырья...
Процедура выполнения команд. Рабочий цикл процессора: Функционирование процессора в основном состоит из повторяющихся рабочих циклов, каждый из которых соответствует...
Методика измерений сопротивления растеканию тока анодного заземления: Анодный заземлитель (анод) – проводник, погруженный в электролитическую среду (грунт, раствор электролита) и подключенный к положительному...
Интересное:
Национальное богатство страны и его составляющие: для оценки элементов национального богатства используются...
Отражение на счетах бухгалтерского учета процесса приобретения: Процесс заготовления представляет систему экономических событий, включающих приобретение организацией у поставщиков сырья...
Наиболее распространенные виды рака: Раковая опухоль — это самостоятельное новообразование, которое может возникнуть и от повышенного давления...
Дисциплины:
![]() |
![]() |
5.00
из
|
Заказать работу |
|
|
Published August 2014
The recent filing of a new JSR for an MVC 1.0 framework in Java EE 8 [1] calls for some clarification on how that JSR relates to JSF. This document aims to reassure the JSF user community about our continued commitment to evolving JSF and to clarify the complementary usages of these two view technologies. Joshua Wilson beat me to the punch with a similar blog entry, which is also well worth reading.
First, let’s get one thing straight. Java EE already has an MVC framework: JSF. Why do we need another one? Isn’t this overkill? The answer lies in a subtle distinction between different kinds of MVC. For the purposes of this article, we consider two kinds of MVC: UI component oriented MVC and action oriented MVC. There are other kinds of MV* concepts out there, but let’s stick with just these two. After looking at these two different styles of MVC, the article explains the rationale for filing a separate JSR for MVC 1.0 and explains how this new specification will relate to the next version of JSF.
UI Component Oriented MVC == JSF
Figure 1: UI Component Oriented MVC as Exposed in JSF
With JSF, the model is CDI, the View is your Facelet pages, and the controller is the JSF lifecycle, as shown in Figure 1. One hallmark of UI component oriented MVC is the emphasis it places on the concept of “inversion of control (IoC)”. JSF takes this concept into the view tier, allowing you to define little bits of code for your view related concerns (conversion, validation, events). This, along with the existing IoC provided by CDI, means that JSF is much more about setting up the pieces and letting the framework do the rest (including managing the view state) than it is about managing the request/response flow of the underlying HTTP.
The following stock symbol lookup code example illustrates the extreme IoC in component oriented MVC by emphasizing the “little bits of code for your view related concerns” concept. First the view: index.xhtml
<!DOCTYPE html><html xmlns="http://www.w3.org/1999/xhtml" xmlns:f="http://xmlns.jcp.org/jsf/core" xmlns:jsf="http://xmlns.jcp.org/jsf"><head jsf:id="head"> <title>Symbol lookup</title></head> <body jsf:id="body"> <form jsf:id="form"> <p>Enter symbol: <input name="textField" type="text" jsf:value="#{bean.symbol}" /> <input type="submit" jsf:id="submitButton" value="submit" jsf:actionListener="#{bean.actionListener}"> <f:ajax execute="@form" render="@form" /> </input> </p> <div jsf:rendered="#{not empty bean.info}"> <p>Info for #{bean.symbol}: #{bean.info}.</p> </div> </form> </body> </html>
This is a simple HTML page instrumented with JSF components. The symbol to lookup is entered into the text field with name='textField'. The button to submit the input with type='submit'. It references an actionListener method. This is where you put your code that looks up the info for symbol. This could interface with a database, a web service, or whatever you like. Note the <f:ajax /> component inside the form’s button. This causes the button to be ajaxified, allowing the form submission and page update to automatically happen over XmlHttpRequest. Finally, there is a conditionally rendered div that displays the result of the lookup.
|
The other portion of code is in the Java class referenced by the #{bean} EL expressions. This is class Bean.java.
import java.io.Serializable; import javax.enterprise.context.RequestScoped; import javax.inject.Named; @Named @RequestScoped public class Bean implements Serializable private String symbol; public String getSymbol() { return symbol; } public void setSymbol(String symbol) { this.symbol = symbol; } public void actionListener() { // Poll stock ticker service and get the data for the requested symbol. this.info= "" + System.currentTimeMillis(); } private String info; public String getInfo() { return info; } }This really is just a simple Java object with a read/write JavaBeans property called symbol, a read only JavaBeans property called info and a method called actionListener().JSF handles all the boilerplate mechanics of the controller. The app will deploy easily to any Java EE 7 container, such as GlassFish 4.0. The default URL after deployment is <http://localhost:8080/symbol/faces/index.xhtml> This simple example is hosted at <https://bitbucket.org/edburns/jsf-symbol>. The example includes two additional files not shown here, one of them the zero-byte marker file: faces-config.xml, the other the Maven pom.xml.
UI component oriented MVC is useful when you are ok with hiding the HTML/CSS/JS and HTTP from the page author. The page author is more about composing UIs from pre-built components and putting in little bits of code here and there to integrate it with the model tier. With this approach, you are indeed placing a lot of trust in the container, but you get a very simple and rapid development model in return.
The benefits of this approach start to diminish when requirements dictate the need to access the lower level HTTP request/response cycle and when more control is desired over the rendering and behavior of the HTML/CSS/JS comprising the UI. The HTML5 Friendly Markup feature of JSF 2.2 mitigates this particular concern somewhat, but there is no getting around the fact that component frameworks look at the world through component colored glasses. These problems have lead to some to label JSF as “too sticky”, but it must be said that this stickiness is a side effect of IoC and dependency injection in general. This stickiness also gets in the way when trying to combine JSF with other technologies without regard for how JSF operates. Finally, for one who is already used to the request/response style of programming, such as those coming from a PHP or Struts background, the learning curve for JSF requires changing the way one thinks about web app development. Such a mindshift is often perceived as not worth the effort. (Your author humbly disagrees.)
Action Oriented MVC == MVC 1.0
In contrast to UI component oriented MVC, the kind of MVC being advocated in MVC 1.0 can be called “action oriented MVC”. This style is shown in Figure 2 and was popularized in frameworks such as Apache Struts and Spring MVC.
|
Figure 2: Action Oriented MVC
The controller dispatches to a specific action, based on information in the request. Each action does a specific thing to transform the request and take action on it, possibly updating the model tier. This approach does not try to hide the request/response model of the underlying HTTP, and it also says absolutely nothing about the specifics of the HTML/CSS/JS comprising the UI.
Though the API for MVC 1.0 is wide open, one piece of prior art that will certainly influence its design is the Jersey MVC system. The Jersey source code includes numerous examples, including the Bookstore Webap, which is excerpted here. Again, we start with the view, this time a simple JSP page.
<%@page contentType="text/html"%><%@page pageEncoding="UTF-8"%> <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <style type="text/css" media="screen"> @import url(<c:url value="/css/style.css"/>); </style> <title>REST Bookstore Sample</title> </head> <body> <h1>${it.name}</h1> <h2>Item List</h2> <ul> <c:forEach var="i" items="${it.items}"> <li><a href="items/${i.key}/">${i.value.title}</a> </c:forEach> </ul> <h2>Others</h2> <p> <a href="count">count inventory</a> <p> <a href="time">get the system time</a> <p> <a href="jsp/help.jsp">regular resources</a> </p> </body></html>In this approach, the style reference must be manually inserted with the @import statement in the <head> section. If the page used JavaScript, a reference to it must also be inserted. With JSF, these resources would automatically be included by virtue of using a component that required them in the page. In the symbol example above, the usage of <f:ajax /> causes a JavaScript reference to be included in the page. The Bookstore Webapp uses several EL expressions, first in the <h1> element. This bean is placed in scope by the Jersey MVC framework. JSTL iterates over the collection of books exposed by ${it}, in the <c:forEach> element.
The index.jsp is rendered implicitly when a GET request to the root URL of the deployed web app is rendered. This happens due to the JAX-RS @Path("/") annotation on the following code
@Path("/") @Singleton @Template @Produces("text/html;qs=5") @XmlRootElement @XmlAccessorType(XmlAccessType.FIELD) public class Bookstore { private final Map<String, Item> items = new TreeMap<String, Item>(); private String name; public Bookstore() { setName("Czech Bookstore"); getItems().put("1", new Book("Svejk", "Jaroslav Hasek")); getItems().put("2", new Book("Krakatit", "Karel Capek")); getItems().put("3", new CD("Ma Vlast 1", "Bedrich Smetana", new Track[]{ new Track("Vysehrad",180), new Track("Vltava",172), new Track("Sarka",32)})); } @Path("items/{itemid}/") public Item getItem(@PathParam("itemid") String itemid) { Item i = getItems().get(itemid); if (i == null) { throw new NotFoundException(Response.status(Response.Status.NOT_FOUND).entity("Item, " + itemid + ", is not found").build()); } return i; } @GET @Produces({MediaType.APPLICATION_XML, MediaType.TEXT_XML, MediaType.APPLICATION_JSON}) public Bookstore getXml() { return this; } public long getSystemTime() { return System.currentTimeMillis(); } public Map<String, Item> getItems() { return items; } public String getName() { return name; } public void setName(String name) { this.name = name; } }Note the manual rendering of HTML anchors in index.jsp. The href of those anchors refers to a RESTful URL that is backed by the getItem() method in the Bookstore.java class. This illustrates the characteristic of action oriented MVC of having to manually deal with the “what happens when the user clicks something in the UI” machinery. With component oriented MVC, all of that is handled by the framework.
|
The Bookstore Webapp runs on GlassFish 4.0 and the source code is available in the Jersey repo on GitHub: <https://github.com/jersey/jersey.git>.
This approach is preferred to a component oriented MVC approach when a greater degree of control over the request/response is required. Web designers may favor this approach because they view the abstraction provided by a component oriented MVC as just getting in the way when what they really want to do is control the HTML/CSS/JS themselves. Finally, this approach is a great fit for REST because of its tight association with the semantics of HTTP.
Conclusion
The decision to include MVC 1.0 in Java EE 8, while continuing to enhance and support JSF, was taken based on community feedback from many sources, including the Java EE 8 Developer Survey [2]. Given the clear community preference to do MVC, the question became: in what part of Java EE should the work be done? Several options were considered before deciding on filing a separate JSR. The most obvious choice was to do the work in the JSF JSR. One approach would be to have an alternate Lifecycle implementation. This would minimize developer confusion from the perspective of overall Java EE. On the other hand, this approach would scare away people who wouldn’t go anywhere near JSF due to its perceived complexity and steeper learning curve, even if the association was only organizational. Another choice was to do the work within the JAX-RS JSR. JAX-RS is a good fit for action oriented MVC because it is inherently is close to HTTP and therefore inherently action oriented. A downside for this option is that it’s impossible to do server side MVC, even the action oriented variety, without introducing some level of statefulness to the system. Pure RESTafarians abhor state, and thus would oppose polluting JAX-RS with statefulness. The last option, of having a separate JSR has the benefit of not scaring people away by association with JSF. The confusion of “which one should I use” is still a possibility, but hopefully this article and the work itself will clear that up.
Finally, don’t pay too much attention to which JSR is hosting the work. It’s better to look at Java EE as a cohesive full stack solution that has both styles built in. These two view technologies will share several key components:
· Use of CDI as the model tier
· Use of Bean Validation as the validation layer
· Use of Expression Language as the glue between the view and the model
· Use of Facelets and/or JSP as the required supported view declaration languages.
The MVC 1.0 JSR will also explore using Faces Flows and/or Flash scope and will likely share elements of JAX-RS.
On the JSF side, in addition to whatever changes are necessary to support MVC 1.0, JSF 2.3 is a community focused release. Topics to be addressed include ajax method invocation, numerous clean up and clarifications, and community requested features such as better support for Twitter Bootstrap, jQuery and Foundation.
About the Author
Ed Burns is currently the spec lead for JavaServer Faces, a topic on which Ed recently co-authored a book for McGraw Hill. Burns is a Consulting Member of the Technical Staff at Oracle. He has worked on a wide variety of client and server side web technologies since 1994, including NCSA Mosaic, Mozilla, the Sun Java Plugin, Jakarta Tomcat and, most recently JavaServer Faces. Ed is an experienced international conference speaker, with consistently high attendence numbers and ratings at JavaOne, JAOO, JAX, W-JAX, No Fluff Just Stuff, JA-SIG, The Ajax Experience, and Java and Linux User Groups.
|
References
[1] https://jcp.org/en/jsr/detail?id=371 [2] https://blogs.oracle.com/ldemichiel/entry/results_from_the_java_eeFollow us on Facebook, Twitter, and the Oracle Java Blog.
GUI Architectures
<--Назадк MVC
There have been many different ways to organize the code for a rich client system. Here I discuss a selection of those that I feel have been the most influential and introduce how they relate to the patterns.
18 July 2006
Martin Fowler
Translations: Greek
This is part of the Further Enterprise Application Architecture development writing that I was doing in the mid 2000’s. Sadly too many other things have claimed my attention since, so I haven’t had time to work on them further, nor do I see much time in the foreseeable future. As such this material is very much in draft form and I won’t be doing any corrections or updates until I’m able to find time to work on it again.
Graphical user interfaces have become a familiar part of our software landscape, both as users and as developers. Looking at it from a design perspective they represent a particular set of problems in system design - problems that have led to a number of different but similar solutions.
My interest is identifying common and useful patterns for application developers to use in rich-client development. I've seen various designs in project reviews and also various designs that have been written in a more permanent way. Inside these designs are the useful patterns, but describing them is often not easy. Take Model-View-Controller as an example. It's often referred to as a pattern, but I don't find it terribly useful to think of it as a pattern because it contains quite a few different ideas. Different people reading about MVC in different places take different ideas from it and describe these as 'MVC'. If this doesn't cause enough confusion you then get the effect of misunderstandings of MVC that develop through a system of Chinese whispers.
In this essay I want to explore a number of interesting architectures and describe my interpretation of their most interesting features. My hope is that this will provide a context for understanding the patterns that I describe.
To some extent you can see this essay as a kind of intellectual history that traces ideas in UI design through multiple architectures over the years. However I must issue a caution about this. Understanding architectures isn't easy, especially when many of them change and die. Tracing the spread of ideas is even harder, because people read different things from the same architecture. In particular I have not done an exhaustive examination of the architectures I describe. What I have done is referred to common descriptions of the designs. If those descriptions miss things out, I'm utterly ignorant of that. So don't take my descriptions as authoritative. Furthermore there are things I've left out or simplified if I didn't think they were particularly relevant. Remember my primary interest is the underlying patterns, not in the history of these designs.
(There is something of an exception here, in that I did have access to a running Smalltalk-80 to examine MVC. Again I wouldn't describe my examination of it as exhaustive, but it did reveal things that common descriptions of it failed to - which even further makes me cautious about descriptions of other architectures that I have here. If you are familiar with one of these architectures and you see I have something important that is incorrect and missing I'd like to know about it. I also think that a more exhaustive survey of this territory would be a good object of academic study.)
Forms and Controls
I shall begin this exploration with an architecture that is both simple and familiar. It doesn't have a common name, so for the purposes of this essay I shall call it "Forms and Controls". It's a familiar architecture because it was the one encouraged by client-server development environments in the 90's - tools like Visual Basic, Delphi, and Powerbuilder. It continues to be commonly used, although also often vilified by design geeks like me.
To explore it, and indeed the other architectures, I'll use a common example. In New England, where I live, there is a government program that monitors the amount of ice-cream particulate in the atmosphere. If the concentration is too low, this indicates that we aren't eating enough ice-cream - which poses a serious risk to our economy and public order. (I like to use examples that are no less realistic as you usually find in books like this.)
|
To monitor our ice-cream health, the government has set up monitoring stations all over the New England states. Using complex atmospheric modeling the department sets a target for each monitoring station. Every so often staffers go out on an assessment where they go to various stations and note the actual ice-cream particulate concentrations. This UI allows them to select a station, and enter the date and actual value. The system then calculates and displays the variance from the target. The system highlights the variance in red when it is 10% or more below the target, or in green when 5% or more above the target.
Figure 1: The UI I'll use as an example.
As we look at this screen we can see there is an important division as we put it together. The form is specific to our application, but it uses controls that are generic. Most GUI environments come with a hefty bunch of common controls that we can just use in our application. We can build new controls ourselves, and often it's a good idea to do so, but there is still a distinction between generic reusable controls and specific forms. Even specially written controls can be reused across multiple forms.
The form contains two main responsibilities:
· Screen layout: defining the arrangement of the controls on the screen, together with their hierarchic structure with one other.
· Form logic: behavior that cannot be easily programmed into the controls themselves.
Most GUI development environments allow the developer to define screen layout with a graphical editor that allows you to drag and drop the controls onto a space in the form. This pretty much handles the form layout. This way it's easy to setup a pleasing layout of controls on the form (although it isn't always the best way to do it - we'll come to that later.)
The controls display data - in this case about the reading. This data will pretty much always come from somewhere else, in this case let's assume a SQL database as that's the environment that most of these client-server tools assume. In most situations there are three copies of the data involved:
· One copy of data lies in the database itself. This copy is the lasting record of the data, so I call it the record state. The record state is usually shared and visible to multiple people via various mechanisms.
· A further copy lies inside in-memory Record Sets within the application. Most client-server environments provided tools which made this easy to do. This data was only relevant for one particular session between the application and the database, so I call it session state. Essentially this provides a temporary local version of the data that the user works on until they save, or commit it, back to the database - at which point it merges with the record state. I won't worry about the issues around coordinating record state and session state here: I did go into various techniques in [P of EAA].
· The final copy lies inside the GUI components themselves. This, strictly, is the data they see on the screen, hence I call it the screen state. It is important to the UI how screen state and session state are kept synchronized.
Keeping screen state and session state synchronized is an important task. A tool that helped make this easier was Data Binding. The idea was that any change to either the control data, or the underlying record set was immediately propagated to the other. So if I alter the actual reading on the screen, the text field control effectively updates the correct column in the underlying record set.
In general data binding gets tricky because if you have to avoid cycles where a change to the control, changes the record set, which updates the control, which updates the record set.... The flow of usage helps avoid these - we load from the session state to the screen when the screen is opened, after that any changes to the screen state propagate back to the session state. It's unusual for the session state to be updated directly once the screen is up. As a result data binding might not be entirely bi-directional - just confined to initial upload and then propagating changes from the controls to the session state.
Data Binding handles much of the functionality of a client-sever application pretty nicely. If I change the actual value the column is updated, even changing the selected station alters the currently selected row in the record set, which causes the other controls to refresh.
Much of this behavior is built in by the framework builders, who look at common needs and make it easy to satisfy them. In particular this is done by setting values, usually called properties, on the controls. The control binds to a particular column in a record set by having its column name set through a simple property editor.
Using data binding, with the right kind of parameterization, can take you a long way. However it can't take you all the way - there's almost always some logic that won't fit with the parameterization options. In this case calculating the variance is an example of something that doesn't fit in this built in behavior - since it's application specific it usually lies in the form.
In order for this to work the form needs to be alerted whenever the value of the actual field changes, which requires the generic text field to call some specific behavior on the form. This is a bit more involved than taking a class library and using it through calling it as Inversion of Control is involved.
There are various ways of getting this kind of thing to work - the common one for client-server toolkits was the notion of events. Each control had a list of events it could raise. Any external object could tell a control that it was interested in an event - in which case the control would call that external object when the event was raised. Essentially this is just a rephrasing of the Observer pattern where the form is observing the control. The framework usually provided some mechanism where the developer of the form could write code in a subroutine that would be invoked when the event occurred. Exactly how the link was made between event and routine varied between platform and is unimportant for this discussion - the point is that some mechanism existed to make it happen.
Once the routine in the form has control, it can then do whatever is needed. It can carry out the specific behavior and then modify the controls as necessary, relying on data binding to propagate any of these changes back to the session state.
This is also necessary because data binding isn't always present. There is a large market for windows controls, not all of them do data binding. If data binding isn't present then it's up to the form to carry out the synchronization. This could work by pulling data out of the record set into the widgets initially, and copying the changed data back to the record set when the save button was pressed.
Let's examine our editing of the actual value, assuming that data binding is present. The form object holds direct references to the generic controls. There'll be one for each control on the screen, but I'm just interested in the actual, variance, and target fields here.
Figure 2: Class diagram for forms and controls
The text field declares an event for text changed, when the form assembles the screen during initialization it subscribes itself to that event, binding it a method on itself - here actual_textChanged.
Figure 3: Sequence diagram for changing a genre with forms and controls.
When the user changes the actual value, the text field control raises its event and through the magic of framework binding the actual_textChanged is run. This method gets the text from the actual and target text fields, does the subtraction, and puts the value into the variance field. It also figures out what color the value should be displayed with and adjusts the text color appropriately.
We can summarize the architecture with a few soundbites:
· Developers write application specific forms that use generic controls.
· The form describes the layout of controls on it.
· The form observes the controls and has handler methods to react to interesting events raised by the controls.
· Simple data edits are handled through data binding.
· Complex changes are done in the form's event handling methods.
Model View Controller
Probably the widest quoted pattern in UI development is Model View Controller (MVC) - it's also the most misquoted. I've lost count of the times I've seen something described as MVC which turned out to be nothing like it. Frankly a lot of the reason for this is that parts of classic MVC don't really make sense for rich clients these days. But for the moment we'll take a look at its origins.
As we look at MVC it's important to remember that this was one of the first attempts to do serious UI work on any kind of scale. Graphical User Interfaces were not exactly common in the 70's. The Forms and Controls model I've just described came after MVC - I've described it first because it's simpler, not always in a good way. Again I'll discuss Smalltalk 80's MVC using the assessment example - but be aware that I am taking a few liberties with the actual details of Smalltalk 80 to do this - for start it was a monochrome system.
At the heart of MVC, and the idea that was the most influential to later frameworks, is what I call Separated Presentation. The idea behind Separated Presentation is to make a clear division between domain objects that model our perception of the real world, and presentation objects that are the GUI elements we see on the screen. Domain objects should be completely self contained and work without reference to the presentation, they should also be able to support multiple presentations, possibly simultaneously. This approach was also an important part of the Unix culture, and continues today allowing many applications to be manipulated through both a graphical and command-line interface.
In MVC, the domain element is referred to as the model. Model objects are completely ignorant of the UI. To begin discussing our assessment UI example we'll take the model as a reading, with fields for all the interesting data upon it. (As we'll see in a moment the presence of the list box makes this question of what is the model rather more complex, but we'll ignore that list box for a little bit.)
In MVC I'm assuming a Domain Model of regular objects, rather than the Record Setnotion that I had in Forms and Controls. This reflects the general assumption behind the design. Forms and Controls assumed that most people wanted to easily manipulate data from a relational database, MVC assumes we are manipulating regular Smalltalk objects.
The presentation part of MVC is made of the two remaining elements: view and controller. The controller's job is to take the user's input and figure out what to do with it.
At this point I should stress that there's not just one view and controller, you have a view-controller pair for each element of the screen, each of the controls and the screen as a whole. So the first part of reacting to the user's input is the various controllers collaborating to see who got edited. In this case that's the actuals text field so that text field controller would now handle what happens next.
Figure 4: Essential dependencies between model, view, and controller. (I call this essential because in fact the view and controller do link to each other directly, but developers mostly don't use this fact.)
Like later environments, Smalltalk figured out that you wanted generic UI components that could be reused. In this case the component would be the view-controller pair. Both were generic classes, so needed to be plugged into the application specific behavior. There would be an assessment view that would represent the whole screen and define the layout of the lower level controls, in that sense similar to a form in Forms and Controllers. Unlike the form, however, MVC has no event handlers on the assessment controller for the lower level components.
Figure 5: Classes for an MVC version of an ice-cream monitor display
The configuration of the text field comes from giving it a link to its model, the reading, and telling it what what method to invoke when the text changes. This is set to '#actual:' when the screen is initialized (a leading '#' indicates a symbol, or interned string, in Smalltalk). The text field controller then makes a reflective invocation of that method on the reading to make the change. Essentially this is the same mechanism as occurs forData Binding, the control is linked to the underlying object (row) and told which method (column) it manipulates.
Figure 6: Changing the actual value for MVC.
So there is no overall object observing low level widgets, instead the low level widgets observe the model, which itself handles many of the decision that would be made by the form. In this case, when it comes to figuring out the variance, the reading object itself is the natural place to do that.
Observers do occur in MVC, indeed it's one of the ideas credited to MVC. In this case all the views and controllers observe the model. When the model changes, the views react. In this case the actual text field view is notified that the reading object has changed, and invokes the method defined as the aspect for that text field - in this case #actual - and sets its value to the result. (It does something similar for the color, but this raises its own specters that I'll get to in a moment.)
You'll notice that the text field controller didn't set the value in the view itself, it updated the model and then just let the observer mechanism take care of the updates. This is quite different to the forms and controls approach where the form updates the control and relies on data binding to update the underlying record-set. These two styles I describe as patterns: Flow Synchronization and Observer Synchronization. These two patterns describe alternative ways of handling the triggering of synchronization between screen state and session state. Forms and Controls do it through the flow of the application manipulating the various controls that need to be updated directly. MVC does it by making updates on the model and then relying of the observer relationship to update the views that are observing that model.
Flow Synchronization is even more apparent when data binding isn't present. If the application needs to do synchronization itself, then it was typically done at important point in the application flow - such as when opening a screen or hitting the save button.
One of the consequences of Observer Synchronization is that the controller is very ignorant of what other widgets need to change when the user manipulates a particular widget. While the form needs to keep tabs on things and make sure the overall screen state is consistent on a change, which can get pretty involved with complex screens, the controller in Observer Synchronization can ignore all this.
This useful ignorance becomes particularly handy if there are multiple screens open viewing the same model objects. The classic MVC example was a spreadsheet like screen of data with a couple of different graphs of that data in separate windows. The spreadsheet window didn't need to be aware of what other windows were open, it just changed the model and Observer Synchronization took care of the rest. With Flow Synchronization it would need some way of knowing which other windows were open so it tell them to refresh.
While Observer Synchronization is nice it does have a downside. The problem with Observer Synchronization is the core problem of the observer pattern itself - you can't tell what is happening by reading the code. I was reminded of this very forcefully when trying to figure out how some Smalltalk 80 screens worked. I could get so far by reading the code, but once the observer mechanism kicked in the only way I could see what was going on was via a debugger and trace statements. Observer behavior is hard to understand and debug because it's implicit behavior.
While the different approaches to synchronization are particularly noticeable from looking at the sequence diagram, the most important, and most influential, difference is MVC's use of Separated Presentation. Calculating the variance between actual and target is domain behavior, it is nothing to do with the UI. As a result following Separated Presentation says we should place this in the domain layer of the system - which is exactly what the reading object represents. When we look at the reading object, the variance feature makes complete sense without any notion of the user interface.
At this point, however, we can begin to look at some complications. There's two areas where I've skipped over some awkward points that get in the way of MVC theory. The first problem area is to deal with setting the color of the variance. This shouldn't really fit into a domain object, as the color by which we display a value isn't part of the domain. The first step in dealing with this is to realize that part of the logic is domain logic. What we are doing here is making a qualitative statement about the variance, which we could term as good (over by more than 5%), bad (under by more than 10%), and normal (the rest). Making that assessment is certainly domain language, mapping that to colors and altering the variance field is view logic. The problem lies in where we put this view logic - it's not part of our standard text field.
This kind of problem was faced by early smalltalkers and they came up with some solutions. The solution I've shown above is the dirty one - compromise some of the purity of the domain in order to make things work. I'll admit to the occasional impure act - but I try not to make a habit of it.
We could do pretty much what Forms and Controls does - have the assessment screen view observe the variance field view, when the variance field changes the assessment screen could react and set the variance field's text color. Problems here include yet more use of the observer mechanism - which gets exponentially more complicated the more you use it - and extra coupling between the various views.
A way I would prefer is to build a new type of the UI control. Essentially what we need is a UI control that asks the domain for a qualitative value, compares it to some internal table of values and colors, and sets the font color accordingly. Both the table and message to ask the domain object would be set by the assessment view as it's assembling itself, just as it sets the aspect for the field to monitor. This approach could work very well if I can easily subclass text field to just add the extra behavior. This obviously depends on how well the components are designed to enable sub-classing - Smalltalk made it very easy - other environments can make it more difficult.
Figure 7: Using a special subclass of text field that can be configured to determine the color.
The final route is to make a new kind of model object, one that's oriented around around the screen, but is still independent of the widgets. It would be the model for the screen. Methods that were the same as those on the reading object would just be delegated to the reading, but it would add methods that supported behavior relevant only to the UI, such as the text color.
Figure 8: Using an intermediate Presentation Model to handle view logic.
This last option works well for a number of cases and, as we'll see, became a common route for Smalltalkers to follow - I call this a Presentation Model because it's a model that is really designed for and thus part of the presentation layer.
The Presentation Model works well also for another presentation logic problem - presentation state. The basic MVC notion assumes that all the state of the view can be derived from the state of the model. In this case how do we figure out which station is selected in the list box? The Presentation Model solves this for us by giving us a place to put this kind of state. A similar problem occurs if we have save buttons that are only enabled if data has changed - again that's state about our interaction with the model, not the model itself.
So now I think it's time for some soundbites on MVC.
· Make a strong separation between presentation (view & controller) and domain (model) - Separated Presentation.
· Divide GUI widgets into a controller (for reacting to user stimulus) and view (for displaying the state of the model). Controller and view should (mostly) not communicate directly but through the model.
· Have views (and controllers) observe the model to allow multiple widgets to update without needed to communicate directly - Observer Synchronization.
|
|
Общие условия выбора системы дренажа: Система дренажа выбирается в зависимости от характера защищаемого...
Адаптации растений и животных к жизни в горах: Большое значение для жизни организмов в горах имеют степень расчленения, крутизна и экспозиционные различия склонов...
Семя – орган полового размножения и расселения растений: наружи у семян имеется плотный покров – кожура...
Архитектура электронного правительства: Единая архитектура – это методологический подход при создании системы управления государства, который строится...
© cyberpedia.su 2017-2024 - Не является автором материалов. Исключительное право сохранено за автором текста.
Если вы не хотите, чтобы данный материал был у нас на сайте, перейдите по ссылке: Нарушение авторских прав. Мы поможем в написании вашей работы!